Post by ridoy06 on Jun 8, 2024 3:14:34 GMT -5
The Power of Jsoup: A Comprehensive Guide for Web Scraping
In today's digital age, the ability to extract data from websites quickly and efficiently is essential for many industries. Whether you are a marketing professional looking to gather insights on consumer behavior, a researcher in need of information for a study, or a developer trying to automate tasks, web scraping tools like Jsoup can be invaluable. In this article, we will dive deep into the world of Jsoup, a Java library that makes web scraping a breeze.
What is Jsoup?
Jsoup is a Java library that allows you to parse HTML documents, extract data, and usa phone number manipulate the contents of a webpage. It provides a simple and flexible API for working with HTML elements, attributes, and text. With Jsoup, you can easily navigate through the structure of a webpage, select specific elements based on CSS selectors, and extract data for further analysis.
How does Jsoup work?
Jsoup works by downloading the HTML content of a webpage and parsing it into a Document object, which represents the entire document. You can then traverse the document tree, select elements using CSS selectors, and extract the desired data. Jsoup also provides methods for cleaning and sanitizing HTML, handling encoding issues, and working with forms.
Why use Jsoup for web scraping?
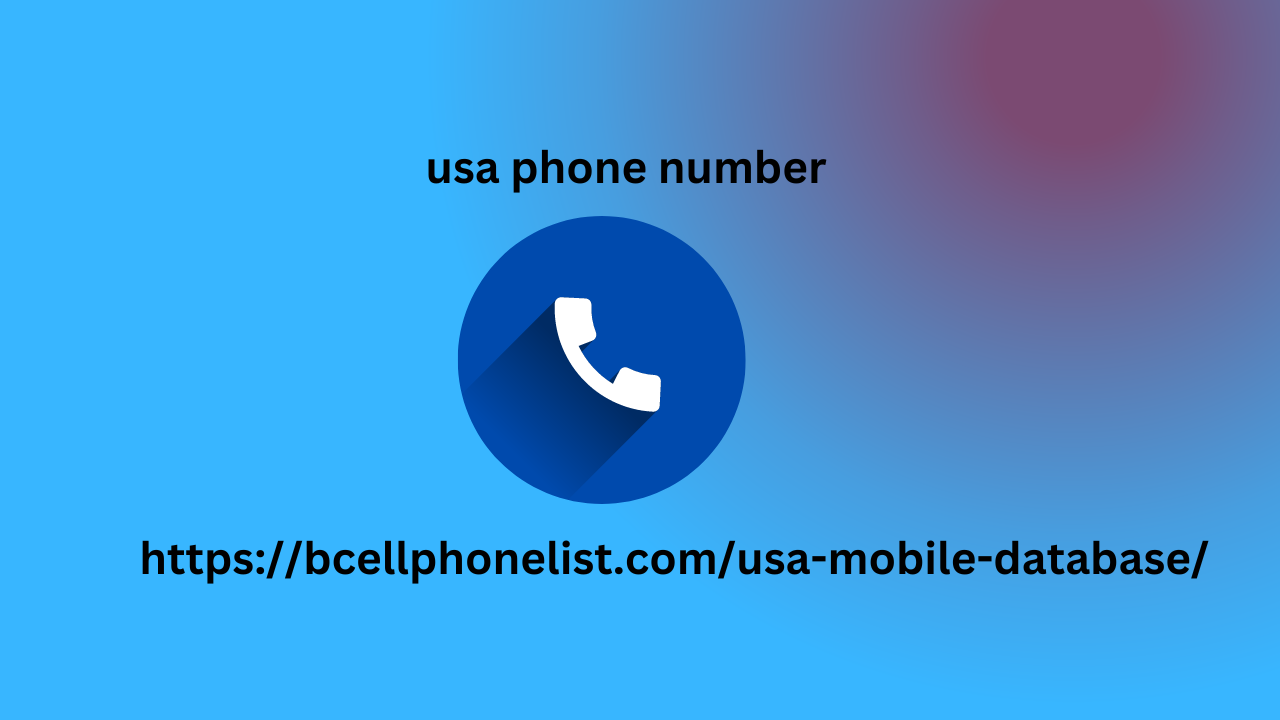
Ease of use: Jsoup's API is intuitive and easy to learn, making it ideal for beginners and experienced developers alike.
Flexibility: Jsoup allows you to extract data from different types of websites, regardless of their complexity.
Performance: Jsoup is optimized for speed and efficiency, making it a reliable choice for scraping large volumes of data.
Community support: Jsoup has a vibrant community of developers who regularly contribute to the library and provide support to users.
Getting started with Jsoup
To start using Jsoup for web scraping, you will need to include the Jsoup library in your Java project. You can download the latest version of Jsoup from the official website or include it as a Maven dependency. Once you have added Jsoup to your project, you can start writing code to extract data from websites.
Example code snippet:
import org.jsoup.*;
import org.jsoup.nodes.*;
public class WebScraper {
public static void main(String[] args) throws Exception {
String url = "https://www.example.com";
Document doc = Jsoup.connect(url).get();
Element title = doc.select("title").first();
System.out.println("Title: " + title.text());
Elements links = doc.select("a[href]");
for (Element link : links) {
System.out.println("Link: " + link.attr("href"));
}
}
}
In this code snippet, we create a simple web scraper that fetches the title of a webpage and the URLs of all links on the page. Jsoup's connect() method is used to download the webpage, and the select() method is used to extract elements based on CSS selectors.
Best practices for web scraping with Jsoup
Respect robots.txt: Before scraping a website, make sure to check its robots.txt file to see if web scraping is allowed.
Use headers: Set a user-agent header in your requests to identify your scraper and avoid being blocked by websites.
Be polite: Avoid sending too many requests to a website in a short period to prevent overloading the server.
Handle errors: Implement error handling in your code to gracefully deal with network issues, timeouts, and other exceptions.
Conclusion
In conclusion, Jsoup is a powerful tool for web scraping that can help you extract data from websites with ease. Whether you are a beginner or an experienced developer, Jsoup's simple API and robust features make it a valuable addition to your toolkit. By following best practices and being respectful of websites' terms of service, you can leverage Jsoup to gather valuable insights and automate tasks efficiently.
Meta Description: Learn how to use Jsoup for web scraping in English, with step-by-step examples and best practices for extracting data from websites. Master the art of web scraping with Jsoup!
In today's digital age, the ability to extract data from websites quickly and efficiently is essential for many industries. Whether you are a marketing professional looking to gather insights on consumer behavior, a researcher in need of information for a study, or a developer trying to automate tasks, web scraping tools like Jsoup can be invaluable. In this article, we will dive deep into the world of Jsoup, a Java library that makes web scraping a breeze.
What is Jsoup?
Jsoup is a Java library that allows you to parse HTML documents, extract data, and usa phone number manipulate the contents of a webpage. It provides a simple and flexible API for working with HTML elements, attributes, and text. With Jsoup, you can easily navigate through the structure of a webpage, select specific elements based on CSS selectors, and extract data for further analysis.
How does Jsoup work?
Jsoup works by downloading the HTML content of a webpage and parsing it into a Document object, which represents the entire document. You can then traverse the document tree, select elements using CSS selectors, and extract the desired data. Jsoup also provides methods for cleaning and sanitizing HTML, handling encoding issues, and working with forms.
Why use Jsoup for web scraping?
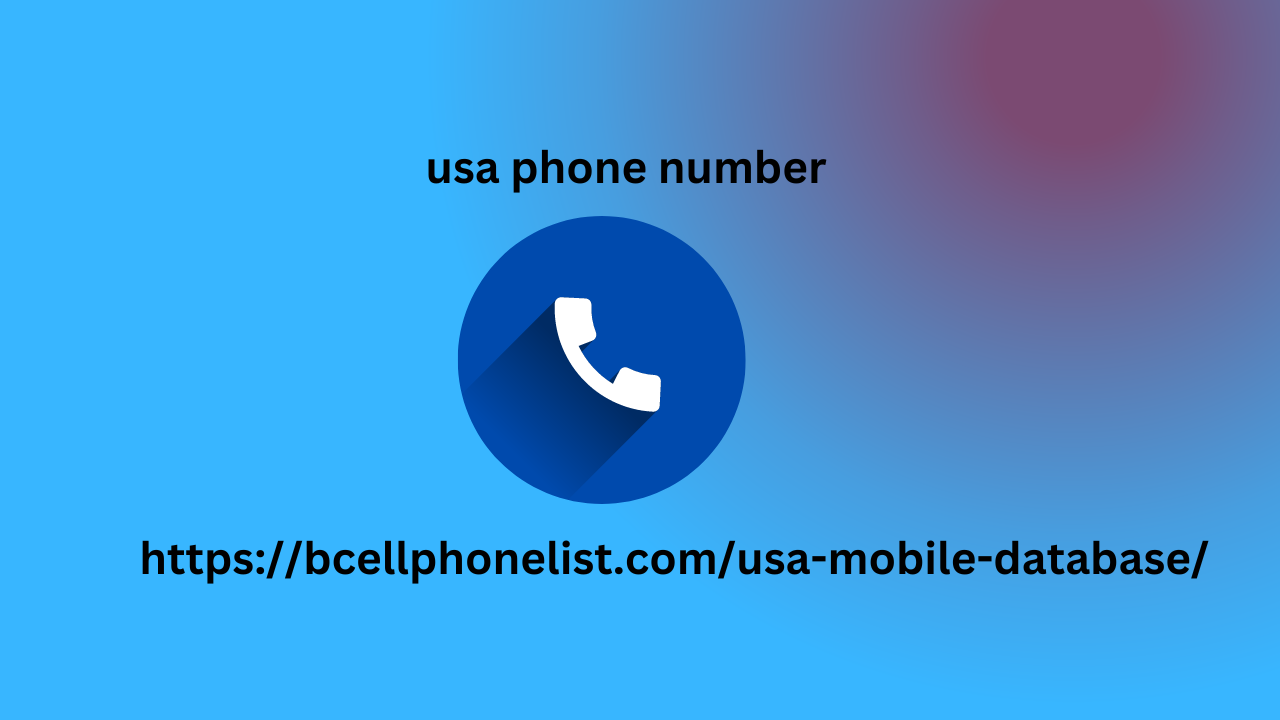
Ease of use: Jsoup's API is intuitive and easy to learn, making it ideal for beginners and experienced developers alike.
Flexibility: Jsoup allows you to extract data from different types of websites, regardless of their complexity.
Performance: Jsoup is optimized for speed and efficiency, making it a reliable choice for scraping large volumes of data.
Community support: Jsoup has a vibrant community of developers who regularly contribute to the library and provide support to users.
Getting started with Jsoup
To start using Jsoup for web scraping, you will need to include the Jsoup library in your Java project. You can download the latest version of Jsoup from the official website or include it as a Maven dependency. Once you have added Jsoup to your project, you can start writing code to extract data from websites.
Example code snippet:
import org.jsoup.*;
import org.jsoup.nodes.*;
public class WebScraper {
public static void main(String[] args) throws Exception {
String url = "https://www.example.com";
Document doc = Jsoup.connect(url).get();
Element title = doc.select("title").first();
System.out.println("Title: " + title.text());
Elements links = doc.select("a[href]");
for (Element link : links) {
System.out.println("Link: " + link.attr("href"));
}
}
}
In this code snippet, we create a simple web scraper that fetches the title of a webpage and the URLs of all links on the page. Jsoup's connect() method is used to download the webpage, and the select() method is used to extract elements based on CSS selectors.
Best practices for web scraping with Jsoup
Respect robots.txt: Before scraping a website, make sure to check its robots.txt file to see if web scraping is allowed.
Use headers: Set a user-agent header in your requests to identify your scraper and avoid being blocked by websites.
Be polite: Avoid sending too many requests to a website in a short period to prevent overloading the server.
Handle errors: Implement error handling in your code to gracefully deal with network issues, timeouts, and other exceptions.
Conclusion
In conclusion, Jsoup is a powerful tool for web scraping that can help you extract data from websites with ease. Whether you are a beginner or an experienced developer, Jsoup's simple API and robust features make it a valuable addition to your toolkit. By following best practices and being respectful of websites' terms of service, you can leverage Jsoup to gather valuable insights and automate tasks efficiently.
Meta Description: Learn how to use Jsoup for web scraping in English, with step-by-step examples and best practices for extracting data from websites. Master the art of web scraping with Jsoup!